Changing a Plane's Diffuse Color and Opacity maps - Solved
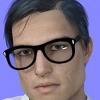
I.m pretty new at DAZ studio scripting but have a background in JS. Im trying to write a script that will increment through a series of diffuse color and opacity strength maps so that I can change the images displayed on the primitive planes. All my diffuse image files end in a number followed by ".png" and my opacity image files end in a number, followed by a "M" and then the ".png". My work so far is:
// Script to increment the Diffuse Color and Opacity values of the material
// On a billboard actor.
//get current selected object
var item = Scene.getPrimarySelection();
var obj = item.getObject();
//now extract the materials for this character
//and extract the diffuse color and Opacity Strength
var oShape = obj.getCurrentShape();
var oMat = oShape.getMaterial( 0 );
var oDiffMat = oMat.findPropertyByLabel('Diffuse Color');
var oOpacMat = oMat.findPropertyByLabel('Opacity Strength');
//Extract the textures for the Billboard and the path to the image files.
var oDiffTex = oDiffMat.getMapValue();
var sDiffPath = oDiffTex.getFilename();
var oOpacTex = oOpacMat.getMapValue();
var sOpacPath = oOpacTex.getFilename();
//Extract the Image index number for the Diffuse Color and increment it by one.
var nIndex = sDiffPath.indexOf('.png',0);
var nDiffLastNum = nIndex - 1;
var sStrNum = sDiffPath.substring(nDiffLastNum,nDiffLastNum+1);
var nDiffImgIndex = sStrNum.valueOf() + 1;
//There should only be six image values.
if (nDiffImgIndex > 6)
{
nDiffImgIndex = 0;
}
//Extract the Image index number for the Opacity and increment it by one.
var nIndex = sOpacPath.indexOf('M.png',0);
var nOpacLastNum = nIndex - 1;
var sStrNum = sOpacPath.substring(nOpacLastNum,nOpacLastNum+1);
var nOpacImgIndex = sStrNum.valueOf() + 1;
//There should only be six image values.
if (nOpacImgIndex > 6)
{
nOpacImgIndex = 0;
}
//Convert the numbers to a strings
var sDiffImgIndex = nDiffImgIndex.toString();
var sOpacImgIndex = nOpacImgIndex.toString();
//Build a new paths
var sTempPath = sDiffPath.substring(0, nDiffLastNum);
var sDiffNewPath = sTempPath.concat(nDiffImgIndex,'.png');
var sTempPath = sOpacPath.substring(0, nOpacLastNum);
var sOpacNewPath = sTempPath.concat(nOpacImgIndex,'M.png');
Now tho I don't see how to write the new paths back to the original materials.... Help!
Comments
use oDiffMat.setMap( sDiffNewPath ) or oOpacMat.setMap( sOpacNewPath )
......
//Build new paths
var sTempPath = sDiffPath.substring(0, nDiffLastNum);
var sDiffNewPath = sTempPath.concat(nDiffImgIndex,".png");
var sTempPath = sOpacPath.substring(0, nOpacLastNum);
var sOpacNewPath = sTempPath.concat(nOpacImgIndex,"M.png");
oDiffMat.setMap( sDiffNewPath );
oOpacMat.setMap( sOpacNewPath );
Although this does not give me an error, it does nothing that I can see, the image is still at index 0 ("D:/DAZ 3D/Studio/My Library/Runtime/textures/Winn/Sex/Solo/Women/Sitting/WhtFemSit00.png") although the paths now read:
sDiffNewPath = "D:/DAZ 3D/Studio/My Library/Runtime/textures/Winn/Sex/Solo/Women/Sitting/WhtFemSit001.png" and
sOpacNewPath = "D:/DAZ 3D/Studio/My Library/Runtime/textures/Winn/Sex/Solo/Women/Sitting/WhtFemSit001M.png"
I even added:
oOpacTex.refresh();
oDiffTex.refresh();
And although that did not cause an error, it didn't work either!
I've changed my script a bit but I still am not getting the Diffuse Color maps or Opacity Strength maps to update. I've tried oDiffMat.setMap() but that didn't work. What do I need to do???
(function NextImage(){
//get current selected object
var item = Scene.getPrimarySelection();
if (item != null)
{
var obj = item.getObject();
//now extract the materials for this character
//and extract the diffuse color and Opacity Strength
var oShape = obj.getCurrentShape();
//get the materials for the billboard item
var oMatBillboard = oShape.getMaterial( 0 );
//Get the properties we're looking for
//from the properties list
var oDiffProp = oMatBillboard.findProperty("Diffuse Color");
var oOpacProp = oMatBillboard.findProperty("Opacity Strength");
//Extract the color maps for the Billboard and
//the path to the map files.
var oDiffTex = oDiffProp.getMapValue();
var sDiffMapPath = oDiffTex.getFilename();
var oOpacTex = oOpacProp.getMapValue();
var sOpacMapPath = oOpacTex.getFilename();
//Extract the Image index number for the
//Diffuse Color and increment it by one.
var nIndex = sDiffMapPath.indexOf(".png",0);
//Make sure it was found.
if (nIndex == -1)
{
MessageBox.warning( qsTr("Improper Diffuse Image!"), qsTr("MyScript"), qsTr("&OK"), qsTr(""));
return;
}
//The last part of image number is one back from the current index
nIndex = nIndex - 1;
//Pull that sub string
var sStrNum = sDiffMapPath.substring(nIndex,nIndex+1);
//Convert it to a number and increment
var nDiffImgIndex = sStrNum.valueOf() + 1;
//There should only be six image values, check if we are at end
//and if so set number to zero.
if (nDiffImgIndex > 6)
{
nDiffImgIndex = 0;
}
//Extract the Image index number for the Opacity and increment it by one.
var nIndex = sOpacMapPath.indexOf("M.png",0);
//Make sure it was found
if (nIndex == -1)
{
MessageBox.warning( qsTr("Improper Opacity Image!"), qsTr("MyScript"), qsTr("&OK"), qsTr(""));
return;
}
//The last part of image number is one back from the current index
nIndex = nIndex - 1;
//Pull that sub string
sStrNum = sOpacMapPath.substring(nIndex,nIndex+1);
//Convert it to a number and increment
var nOpacImgIndex = sStrNum.valueOf() + 1;
//There should only be six image values, check if we are at end
//and if so set number to zero
if (nOpacImgIndex > 6)
{
nOpacImgIndex = 0;
}
//Convert the numbers to strings
var sDiffImgIndex = nDiffImgIndex.toString();
var sOpacImgIndex = nOpacImgIndex.toString();
//Build new paths
var sTempMapPath = sDiffMapPath.substring(0, nIndex-1);
var sDiffNewMapPath = sTempMapPath.concat(".png");
sTempMapPath = sOpacMapPath.substring(0, nIndex-1);
var sOpacNewMapPath = sTempMapPath.concat("M.png");
//print(sDiffNewMapPath);
//print(sOpacNewMapPath);
}
else
{
MessageBox.warning( qsTr("You Must Select a Object in the Scene!"), qsTr("MyScript"), qsTr("&OK"), qsTr(""));
return;
}
})();
Look at the end of the thread here: https://www.daz3d.com/forums/discussion/91931/setting-a-dzimageproperty-value-to-a-new-dztexture-solved
I ran into some issues doing this kind of thing....turns out there are two different cases you have to handle (to be a general solution) but for just Opacity and Diffuse, you could probably hard-code it (i.e., just call oDiffProp.setMap(filename); for example. (I do believe both Opacity and Diffuse Color are DzNumericProperty, not DzImageProperty.)
Sorry, I missed your earlier reply - I would have asked if you were checking that the filename was being correctly generated, via printing it out or the debugger.
I did look at the thread and I changed my script acccordingly except I limited the scope to just changing the Diffuse Color, It seems to be working now! I will now add code to also change the Opacity Strength and see if that works.
After looking through the code in the above example, I made some changes to my code and I now have a working script. Thanks a lot for the input from Richard Haseltine and hphoenix , I really appreciate the feedback!