Problem with clamping size of DzDialog [solved]

There is some strange behavior with DzDialog when it comes to controlling the window dimensions.
I have a dialog window I built in Qt Designer. I found out the hard way that I can't load a dialog from .ui, but instead a widget, then need to create the dialog in script, attach a top-level layout, then attach the widget as a child. So far, so good, but this isn't the exact problem...
I designed the window to be minimum 600x300, and maximum 1600x300. In Designer it works perfectly no matter what, whether it is a Dialog or a Widget.
Now I create the dialog, load the.ui, and add the goods. It appears to be ok, but the dialog has no limits, in spite of setting them explicitly (more about this in a moment)
This is the code, in its own script file, nothing else:
function doDlg(){ var uLoader = new DzUiLoader(); var wDialog = new DzDialog(); var wLayout = new DzGridLayout( wDialog ); var wgt = uLoader.load("C:/...<snipped>.../dlg.ui", wDialog); wLayout.addWidget ( wgt, 0, 0, 0, 0 ); wDialog.maximumSize = ( 1600,300 ); wDialog.exec(); };
The order of building the dialog and adding top-level layout is as specified in docs. The widget that is loaded from .ui behaves, and respects the designed limits. The DzDialog it inhabits, however, does not.
But wait, it gets better...
If I replace
wDialog.maximumSize = ( 1600,300 );
with
wDialog.setFixedSize( 600, 300 );
THEN, the dialog box is clamped at that size... BUT, ONLY if run and call it from within that script page. No matter if it's in a function, or executing in global scope, it behaves.
HOWEVER, and this is the bite on the butt, if I include this script in my main script and then call doDlg() from there (or even include the script with the dialog in global scope without a function wrapper)... again, the dialog window that appears ignores any size restrictions. The widget loaded inside always does though... and it looks dopey as all heck.
It doesn't matter when I set the fixed size after creating the dialog object, it still behaves as described and setFixedSize is the only restriction parameter it respects (when it does, lol). As it inherits DzWidget, it should and does take all applicable parameters without throwing exceptions. It just doesn't feel like obeying.
Am I doing something wrong with this setup, or is this buggy?
Me right now:
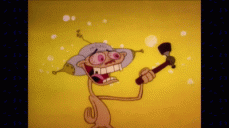
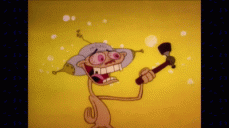
Comments
I can't see MaximumSize as a method or property for any of the objects in the Daz Script documentation. MaxHeight and MaxWidth, however, are properties of the DzWidget object. I don't know if they will do the job.
maximumSize is listed as an exposed member in the QWidget wrapper
http://docs.daz3d.com/doku.php/public/software/dazstudio/4/referenceguide/scripting/api_reference/object_index/widget_q#a_1aa654bd70a1fdb95c10bf8f2bb4a99470
"TODO: Add description".
Checking the QWidget docs
https://doc.qt.io/archives/qt-4.8/qwidget.html#maximumSize-prop
I see maximumSize is a constant, not a method. D'oh!
Access functions:
However, the setters don't appear to be wrapped by DzWidget. Attempting to call wDialog.setMaximumSize( 1600, 300 ) throws TypeError: Result of expression 'wDialog.setMaximumSize' [undefined] is not a function.
And I guess I forgot to mention earlier, maxHeight and maxWidth also have no effect at all. Again, no errors.
Although the Daz Script widgets wrap QWidget they do not inherit from it - you need to use DzWidget.getWidget() to get the QWidget. Also note that you need to pass the values as a Size object, not a pair of values.
Yes, but it's not the widget that is the problem, it is the DzDialog. The widget inside behaves perfectly.
Unfortunately you can't cast the dialog to a DzWidget to call getWidget() (or can you?) ... attempting to call DzDialog.getWidget() gives an error "TypeError: Result of expression 'DzDialog.getWidget' [undefined] is not a function."
Also, I made an error in my post above; I meant "wDialog.MaximumSize( 1600, 300 ) throws TypeError..." I should get more sleep and not post near dawn lol.
Actually, calling wDialog.setFixedSize( 1600, 300 ) works fine, even though the doc does not list it as an inherited member from DzWidget (!). It just seems to be the only function that does though. However, the size is then of course locked, and I really want the user to be able to stretch it out reasonably if they want to see a long line of input without cursor-scrolling in the text input. URLs can get pretty long...
Well thank you so much for the help, but this is still broken. Not a game-ender, but I'm really not going for the bush league look-and-feel. When I get this tool working to my satisfaction and it does the job I need it to do, I intend to publish in the store. I guess I'll just leave it at a fixed size around 1000px wide.
Are you literally calling through the wDialog object? If that isn't a placeholder for explanation then you need to make sure you are calling through your actual dialog object. See http://docs.daz3d.com/doku.php/public/software/dazstudio/4/referenceguide/scripting/api_reference/samples/start#general_interface
DzDialog does inherit DzWidget, so it has access to the members and methods of that (unless overridden). The scripting documents don't repeat features of parent objects, they isntead list the inheritance which needs to be traced back. However, MaximumSize is not a member of DzDialog - all members of Dz objects have a lower case initial letter for one thing - and it is not a function in QDialog or QWidget. DzWidget does have a maximumSize property (which is not a function) - it wraps, but is not itself, the QWidget.maximumSize() and QWidget.setMaximumSize() which are not publicly available.
Hey, thanks for all the help, really. Patience of a Saint :^)
SO, after quite a bit of study on this, I figured out that if you want Nice Things™, you will have to do extra work.
Ultimately, I cannot do what I need to do with my UI by simply craeating it in Designer and loading the .ui, because of the incredible shortcomings of the Dz bindings, and the fact that you only get native Qt out of Designer. The Dz counterparts to Qt widgets are only partially implemented. Kinda makes you wonder why they included Designer at all, if they're going to bundle it with a Playskool implementation of the widgets.
So the long and the short of it is, I must do what I was trying to avoid doing in the first place, and completely write the UI in script. This makes the script page much larger than I would like, but then I can work with pure DzWidgets. I would have lived with a fixed size window as a tradeoff, but then today I came across the Impossible ComboBox scenario. It simply doesn't do anything useful that isn't an available slot, as the useful methods are not wrapped. Therefore I was forced to make a pure Dz UI where I can work directly with the DzComboBox methods.
As for the dialog box size thing, what it turns out to be is: spawn a DzDialog, get the QWidget it wraps, and manipulate that. Not surprisingly, you cannot use most of the Qt methods on the QWidget (and ancestors) via Daz Script, but unexpectedly I found you can indeed assign the Properties directly. I haven't seen it documented, but they are not read-only in this case (I would have expected read-only properties, and setters and getters to change them).
So basically,
Works perfectly.
Designer is mainly for the SDK, which can use its output directly, but it is usable in scripting (and is used in some scripts).
See http://docs.daz3d.com/doku.php/public/software/dazstudio/4/referenceguide/scripting/api_reference/samples/start#educationassistance for examples that use a Designer set up through DzUiLoader or DzUIPopUpWgt and also look in the application folder for
/resources/guide pages/Common/GuidePage.dsa
/resources/tip pages/Common/TipPage.dsa
/resources/Lesson Strips/Common/Popup.dsa
/resources/Lesson Strips/Common/Overview.dsa