How to access DzOpacityManip (How to change opacity on LIE Layers)
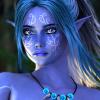
I have a shader preset that uses the Layered Image Editor. I am trying to make a script that will alter the opacity setting on one of the layers. I have been looking at the Daz Script 2 doc and at lots of script examples, but I feel like I'm missing the big picture. I just don't understand how I get to the layer so I can change the opacity. If anyone has any advice I would really appreciate it.
Post edited by parrotdolphin on
Comments
There's some general good info in programming the LIE here. To the point of your question, it looks like image layers have an opacity property as opposed to a setOpacity() method.
I only have a semi-working example right now, or I'd post the entire thing. Aparently if you createLayeredTexture from scratch instead of a file, it defaults to a size of -1 x -1. I did verify that it sets the opacity of the layer, though.
Note:
Prior to 4.6.2.23 you needed to do something like the following (guessing here):
I don't have any way to test it though.
OK, this does what I expect:
The dumpBehavior function is how I find all the things that DAZ doesn't document.
The two images were just gradients that I created in photoshop for testing. They're included here for reference.
Thanks so much Esemwy! I'm going to see how far I can get with your great example. I'm not creating a layered image with my script, I just want it to change the opacity on an existing layer for a selected surface. Hopefully, using dumpBehavior will be enlightening. I need all the enlightenment I can get.
Never mind. This was an erroneous response.
Hmmm....
You can then getLayer(int), etc on oLayeredImage.
Edit:
P.S. Yes, I'm a bit obsessive when it comes to code.
I managed to get the number of layers and change the opacity on the layer I wanted. I did it a bit differently than your latest post. Now I just want to make sure I have the right name on the layer before I change it. So, I'm working on that. You have helped me so much. I've spent hours and hours on this script and without your example, I got nowhere.
I'm glad I could be of help.
When I have my Viewport set to NVIDIA Iray and I run my script to change opacity on a layer, nothing changes. If I set the Viewport to Texture Shaded then I see the change.
When I have my Viewport set to NVIDIA Iray and I go directly into the Layered Image Editor and change the opacity then I see the change.
I looked at the log and noted a difference:
Direct lie update:
Loaded image AC08.jpg
Loaded image F1.jpg
Loaded image G03.jpg
Iray INFO - module:category(IMAGE:IO): 1.0 IMAGE io info : Loading image "C:/Users/Janet/AppData/Roaming/DAZ 3D/Studio4/temp/d16.png", pixel type "Rgba", 1024x1024x1 pixels, 1 miplevel.
Iray INFO - module:category(IRAY:RENDER): 1.0 IRAY rend info : Updating materials.
Update via script:
Loaded image AC08.jpg
Loaded image F1.jpg
Loaded image G03.jpg
Iray INFO - module:category(IRAY:RENDER): 1.0 IRAY rend info : Updating materials.
So, then I thought maybe I have to save the temp layered image. Here is my script so far, but the temp image is never saved and the Iray Veiwport still isn't changing. Any idea what I could do? Note that you'd need a Layered Image with 3 layers to run it.
My workstation is tied up with a render right now, but I'll try to take a look at it in the morning.
That would be wonderful if you have time. Thanks!
OK, I think I see what's going on. The preview issue seems almost, but not quite, opposite from what you describe if you apply the texture to a plane instead of say a cube.
It appears as if Iray, for whatever reason, caches a bit more fervently than the textured shader. If the LIE is allowed to create a new layered image, all is well, if not, Iray stays with the cached texture regardless of what has been written to the file. In other words, all the work you're doing trying to refresh the image cache is being ignored by Iray. I believe if you re-write your script to save the layered image to a new file and then swap the map, it will behave in both. It's ugly, but this is what the LIE does nornally, and this is probably the reason.
Thanks Esemwy. I am trying your suggestion. I tried this code below but it doesn't work. I get a .dsi file but no .png
The pd-001.dsi file is text which says:
I suspect the line, var oLayeredImg = new DzLayeredImage(oTexture); is wrong. But I'm not sure how to make it right.
I'm not having much luck either. Perhaps we can create a new layered texture, then copy the layers, that would do the trick. I've not quite figured that out yet, though.
I think I'll take a break from it for a while, maybe render something, and the answer may come to me.
I was thinking that might work too. I will give it a shot and post back here when it's working or I get stuck again. Thanks again.
Small miracle... I think I'm actually getting somewhere.
It needs some more work though, so I'll post more later.
When the viewport is set to NVIDIA Iray, this code works if I only select one surface. If I select more than one surface and the viewport is set to NVIDIA Iray, the surfaces turn white. If I then go to Texture Shaded in the Viewport, I see the correct changes. Then if I go back to NVIDIA Iray in the Viewport, the surfaces now look correct. So, I don't know. Still missing something... but it's progress. Again, note that you need 3 layers to run this.
Please. Try this, works for me.
You can directly change opacity there's no need to copy it. layer.NeesRefresh() instructs renderer to refresh views.
HTH
Forget it, does not work! It does not refresh the resulting layered image. I'll keep investigating.
Thanks. Yes, that works when I use Texture Shaded in the Viewport, but not when I use NVIDIA Iray in the Viewport. I was trying to get it to update the texture for the Iray preview so that's why I resorted to copying the layers.
Ok, this is working.
Cool. I'm just now getting back to this. Nice to see it solved in my absence. One minor fix, though. Don't know why I didn't see it when I was looking before.
DzLayeredTexture::copyFrom(const DzLayeredTexture*) will perform a deep copy of the object so you don't have to do all the heavy lifting yourself.
Excellent, I think it only needs one additional validation to ensure it is a DzLayeredTexture instance.
I reordered things a bit and modified the surface selection logic. Here's the entire thing.
Once I did all the defensive programming, it made more sense to bring cloneLayeredTexture() inline. I believe the differences in my logic are immaterial.
Thank you both for all the help. This has helped me a lot. :)
Just getting back to this script again...
I am still having the problem where the surface turns white when I run the script while the viewport is set to NVIDIA Iray. This doesn't happen if I run the script from the Script IDE tab, but when I run it out of a folder in the Content Library it does happen. If I create a Custom Action and then run the script from the Scritps menu (on the top tool bar) then it doesn't happen. I have no idea why it works this way. Does anyone have any idea? I would like to be able to run it from a folder in the Content Library.
That's a bug in Daz Studio... From what I understand it's been reported. If you click to something else (like texture) and then click back to NVIDIA Iray it should update the viewport.
Thanks for the info DraagonStorm. Yes, it looks fine if I switch to Texture Shaded and back again.